In this tutorial, we'll learn how we can use Next.js with Strapi and Apollo.
Table of Contents
- Introduction
- Creating a Strapi application using Docker
- Creating a Next.js application
- Integrating the Next.js application with Apollo
- Conclusion
Introduction
In one of my previous articles, I've written about how to get started using Strapi. In this post, we'll be building a newsfeed application using Next.js. The APIs necessary for the Next.js front-end application will be powered by Strapi. We'll also use Apollo as the GraphQL client.
The whole code for the application that we're going to build is available on Github.
Before we proceed, it's better if you have some idea about the following technologies:
I've been using Strapi for quite some time now and it's very easy to get up and running with it within a very short amount of time. It gives us a lot of features out of the box:
- Single types: Create one-off pages that have unique content structure
- Customizable API: With Strapi, you can just hop in your code editor and edit the code to fit your API to your needs.
- Integrations: Strapi supports integrations with Cloudinary, SendGrid, Algolia and others.
- Editor interface: The editor allows you to pull in dynamic blocks of content.
- Authentication: Secure and authorize access to your API with JWT or providers.
Next.js is a very popular React framework. It offers a lot of features like:
- Zero config: Automatic compilation and bundling. Optimized for production from the start.
- Hybrid: SSG and SSR: Pre-render pages at build time (SSG) or request time (SSR) in a single project.
- Incremental Static Generation: Add and update statically pre-rendered pages incrementally after build time.
- TypeScript Support: Automatic TypeScript configuration and compilation.
- Fast Refresh: Automatic TypeScript configuration and compilation.
Apollo is the industry-standard GraphQL implementation, providing the data graph layer that connects modern apps to the cloud. It offers a lot of features like:
- Declarative data fetching: Write a query and receive data without manually tracking loading, error, or network states.
- Reactive data cache: Cut down on network traffic and keep data consistent throughout your application with Apollo Client’s normalized reactive data cache.
- Excellent dev experience: Enjoy cross stack type safety, runtime cache inspectors, and full featured editor integrations to keep you writing applications faster.
- Compatible and adoptable: Use any build setup and any GraphQL API. Drop Apollo Client into any app seamlessly without re-architecting your entire data strategy.
- Designed for modern UIs: Take advantage of modern UI architectures in the web, iOS, and Android ecosystems.
I've created a boilerplate so that you can get up and running with Strapi, Next.js and Apollo quickly. Check out the project on Github.
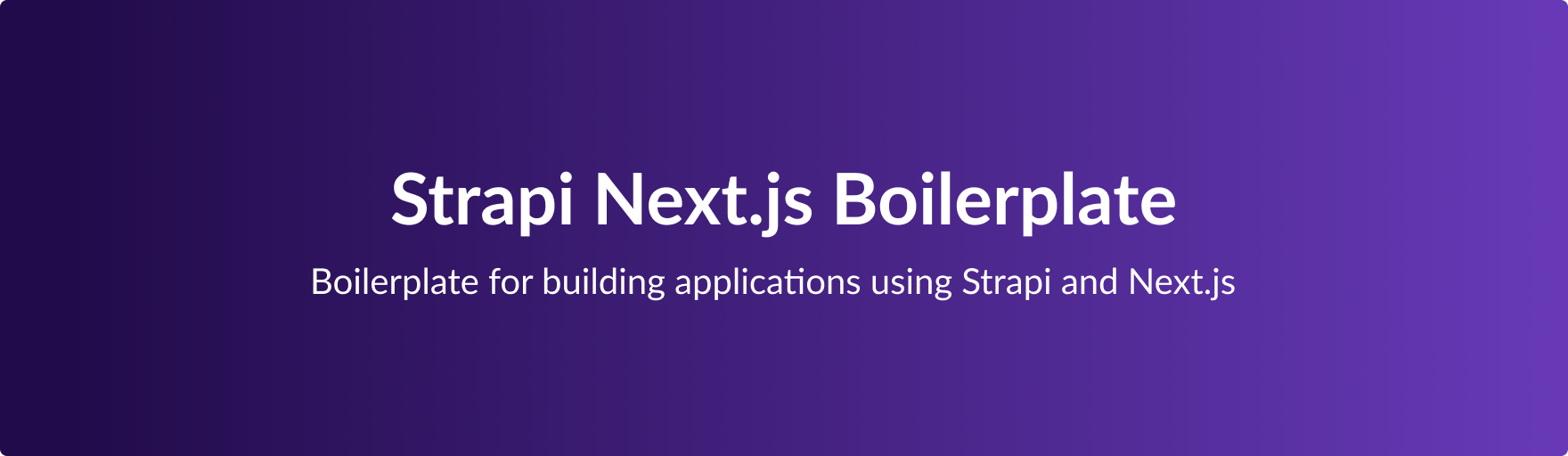
Creating a Strapi application using Docker
Step 1: We need to create a directory named backend
and then download the docker-compose.yml file from the strapi-docker repository. Now, we need to start Docker. Once Docker is up and running, we can go inside the backend
directory and run our Strapi container:
cd backend && docker-compose up
This will pull postgres and strapi/strapi images from Docker Hub. So, it might take some time for this operation to complete. You can refer to this article for more details regarding how to install Strapi using Docker.
Step 2: We'll have to create our first administrator profile. Once, our administrator profile is setup, we should be able to log into the admin panel of Strapi.
Step 3: We'll have to add a new content-type.
Step 4: Install the Strapi GraphQL plugin.
I've already covered most about getting started with Strapi. We're adding links to a previous article in order to keep this tutorial short.
Creating a Next.js application
We can create a new Next.js app using create-next-app, which sets up everything automatically for us:
yarn create next-app
The above command will install all the necessary packages as well a create a new directory based on the application name (which you entered during the setup process).
Integrating the Next.js application with Apollo
In order to integrate Apollo with Next.js, we need to add the required dependencies first:
yarn add @apollo/client graphql
Next, we need to create a new file lib/with-graphql.js
with the following content:
import { ApolloClient, ApolloProvider, InMemoryCache } from "@apollo/client";
const WithGraphQL = ({ children }) => {
const client = new ApolloClient({
uri: "http://localhost:1337/graphql",
cache: new InMemoryCache(),
});
return <ApolloProvider client={client}>{children}</ApolloProvider>;
};
export default WithGraphQL;
Now, we can import this file and wrap any Next.js page where we want to use GraphQL:
import React from "react";
import Page from "components/pages/index";
import WithGraphQL from "lib/with-graphql";
const IndexPage = () => {
return (
<WithGraphQL>
<Page />
</WithGraphQL>
);
};
export default IndexPage;
Now, we can use GraphQL queries and mutations in the components/pages/index.js
file:
import { gql, useQuery } from "@apollo/client";
import { Box, Stack } from "@chakra-ui/core";
import Feed from "components/pages/index/feed";
import React from "react";
const feedsQuery = gql`
query fetchFeeds {
feeds {
id
created_at
body
author {
id
username
}
}
}
`;
const FeedsPageComponent = () => {
const { loading, error, data } = useQuery(feedsQuery);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error :(</p>;
return (
<Stack spacing={8}>
{data.feeds.map(feed => {
return (
<Box key={feed.id}>
<Feed feed={feed} />
</Box>
);
})}
</Stack>
);
};
export default FeedsPageComponent;
Conclusion
In this tutorial, we've learnt how we can integrate Apollo with Next.js and use it with Strapi. I've created a boilerplate so that you can get up and running with Strapi, Next.js and Apollo quickly. Check out the project on Github. Documentation of this project is available here.
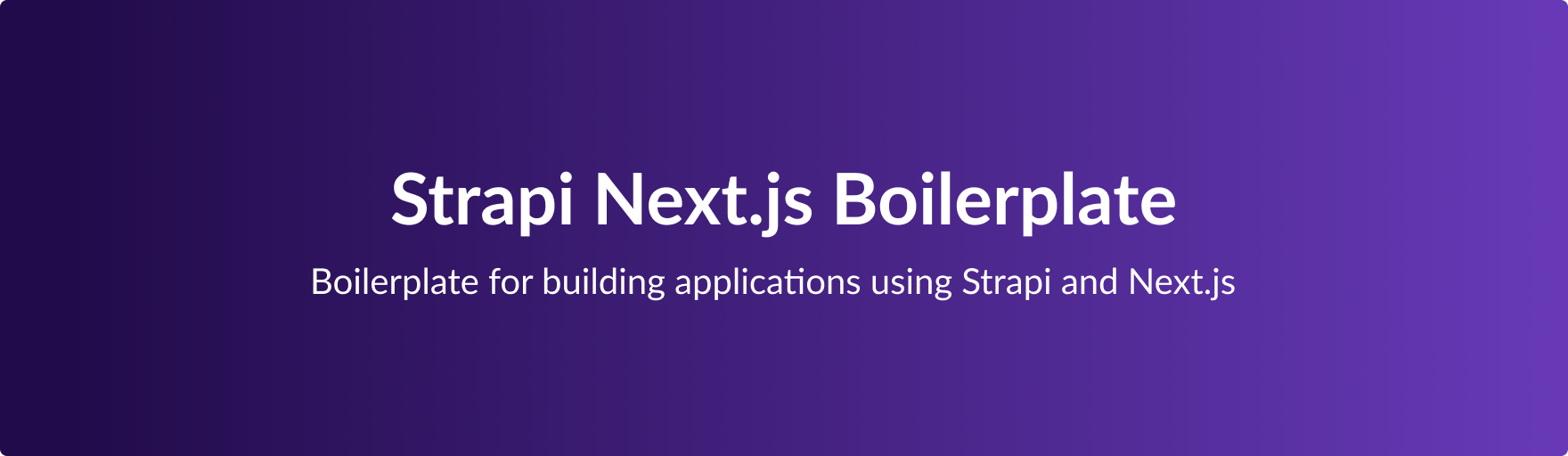